Introduction
This page serves as an introduction to incorporating ACRE into Agent Factory, by enabling agents to reason about conversations in which they are engaged. The language used in the examples is AF-AgentSpeak, although any language that has been integrated into the Common Language Framework should work in a similar fashion.
Download/Install
To use ACRE, you will need:
- The two ACRE JAR files: MAS-ACRE and AF-ACRE (in your classpath).
-
If you wish to see protocol visualisations, you will need the
dot
utility from Graphviz, which must be in your path. Installation instructions can be found here.
Setup
After downloading and installing the components above, in order to keep track of the protocols to be used by the ACRE-enabled agents, you must first load the ACRE Protocol Manager Platform Service in your run configuration.
The following Debug Configuration is adapted from the AF-AgentSpeak user guide. The additional lines necessary to load the ACRE Protocol Manager Platform Service and its debugger addon are highlighted in bold:
import is.lill.acre.debugger.ProtocolManagerServiceInspectorFactory; import is.lill.acre.service.ProtocolManagerPlatformService; import com.agentfactory.agentspeak.interpreter.AgentSpeakArchitectureFactory; import com.agentfactory.agentspeak.debugger.AgentSpeakStateManagerFactory; import com.agentfactory.agentspeak.debugger.inspector.AgentSpeakInspectorFactory; import com.agentfactory.visualiser.DebuggerRunConfiguration; public class HelloDebugConfiguration extends DebuggerRunConfiguration { public String getName() { return "helloworld"; } public String getDomain() { return "ucd.ie"; } public void configure() { addServiceInspectorFactory( new ProtocolManagerServiceInspectorFactory() ); addLanguageSupport(new AgentSpeakInspectorFactory(), new AgentSpeakStateManagerFactory()); super.configure(); addArchitectureFactory(new AgentSpeakArchitectureFactory()); addPlatformService( ProtocolManagerPlatformService.class, ProtocolManagerPlatformService.NAME ); addAgent("Bob", "helloworld.aspeak"); } public static void main(String[] args) { new HelloDebugConfiguration().configure(); } }
The first of these lines is to allow integration of the Protocol Manager into the debugger. When the Agent Factory Debugger is launched, an additional entry is present under the Platform Services section of the left-hand side-pane. The tabs in that debugger panel allow you to see the agents that are bound to the platform service, and also the protocols that have been loaded. Double-clicking on the name of a protocol will result in a diagram being generated in the main section of the screen (requires Graphviz: see above). The debugger is shown in the following screenshot:
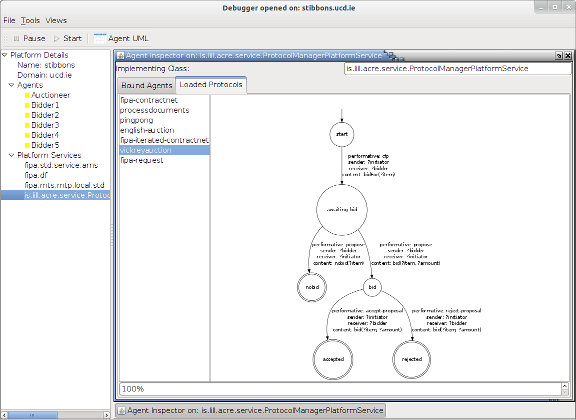
The second additional line in the Debug Configuration loads the Protocol Manager Platform Service itself. Without this, none of the agents will have access to ACRE protocols and the advantages of using ACRE are lost.
If it is desired to make the Protocol Manager aware of a protocol repository on startup, the above line to add the Platform Service may be replaced with:
Properties prop = new Properties(); prop.put( "repository", "http://acre.lill.is/examples" ); addPlatformService( ProtocolManagerPlatformService.class, ProtocolManagerPlatformService.NAME, prop );
Writing Agents Loading the ACRE Module
Loading the ACRE Module
When writing an ACRE-enabled agent, the first requirement is that the ACRE Module be loaded. This serves as the interface between the agent and the ACRE components. It exposes a number of actions to the agent that facilitate easier communication, along with providing information (by way of appropriate beliefs) about the status of ongoing conversations and loaded protocols.
The module is loaded by adding the following line of code to your agent:
module acre -> is.lill.acre.agent.module.ACREModule;
When the module is loaded, it is necessary to initialise it using the init
action. This causes the module to connect to the Protocol Manager Platform Service, so that protocol definitions can be accessed. Once this has occurred, the belief ready(acre)
is adopted to indicate this.
In order to be able to engage in conversations, it is necessary to load some protocols from a protocol repository. This is done by means of the addRepository
action. It is generally a good idea to do this before calling the init
action. This way, the repository URL is supplied to the module before it connects to the Protocol Manager. When this connection is made, the repository is loaded immediately, meaning that when the ready(acre)
belief is adopted, your agent is fully ready to engage in conversations.
It is important to note that each ACRE-enabled agent has access to all protocols that have been loaded by the Protocol Manager. For this reason, it is not necessary for every agent to add the same protocol repository when it connects to the platform service.
A typical commitment rule to initialise the ACRE module is as follows. This is triggered on the first iteration of the agent, when the initialised
event is automatically triggered (this is a core feature of AF-AgentSpeak and is not ACRE-specific).
+initialized : true <- acre.addRepository("http://acre.lill.is"), acre.init;
Starting Conversations
A conversation may be initiated using the start
action. This action requires four parameters, as follows:
- The Protocol Identifier is used to uniquely identify the protocol that the conversation is to follow. Generally, this follows the pattern namespace_name_version. However, for convenience, in smaller systems the name alone can be provided, where it is known that there will not be two protocols with the same name.
-
The Participating Agent is the identifier of the agent with which you wish to converse. If this has previously been added to the address book, then just the name of the agent is required. If not, a full agent identifier must be provided, in the form
agentID(name,addresses(address1[,address2...]))
- The performative of the first message to be sent as part of this conversation.
- The content of the first message to be sent as part of the conversation.
The start
action will return the automatically-generated conversation identifier, so that the conversation may be recorded, reasoned about and referred to later.
As an example, assume that the first task to be carried out is to initiate a conversation with an agent named John
. This conversation should begin a Vickrey Auction using a protocol named vickreyauction
. The following rule may be used for this purpose:
+ready(acre) : true <- acre.addContact(agentID(John,addresses("local:localhost"))), ?cid = acre.start(vickreyauction,John,cfp,bidFor(painting)), .println( ?cid );
Responding to Conversations
Sending a message as part of an active conversation is done by using the advance action. With active conversations, the ACRE Module has recorded the details of the other agent involved in the conversation, regardless of which agent began it.
The advance action requires three parameters:
- The unique identifier of the conversation you wish to advance (this will typically be acquired by using a commitment rule).
- The performative of the message to be sent.
- The content of the message to be sent.
The following is an example of a commitment rule that would be used to advance a conversation:
+conversationAdvanced(?cid,awaiting_bid,?length) : true <- acre.advance(?cid,propose,bid(painting,200));
This rule is triggered by a conversationAdvanced
event, which indicates that the state of the conversation has been changed by the sending or receipt of a message. This belief includes the name of the state that the conversation has been advanced to, as well as the current length of the conversation (i.e. the number of messages that have been exchanged as part of the conversation).
For AF-AgentSpeak agents, it strongly advised to use ACRE events as triggers for commitment rules. Using knowledge percepts as triggers may result in a situation where no event is triggered by AF-AgentSpeak, as the agent's belief base has not changed since the last iteration.
Cancelling Conversations
Either agent involved in a conversation may ask for it to be cancelled at any time. However, the conversation is not considered to be cancelled unless both participants agree. The cancellation process is initiated by using the cancel action, as illustrated below:
+wantToCancel(?cid) : true <- acre.cancel(?cid);
For an agent that receives a request to cancel a conversation, it may reply either by confirming the cancellation (using the confirmCancel
action) or by denying the request (using denyCancel
). The cancellation request itself is indicated by a conversationCancelRequest
event being triggered. This is illustrated below:
+conversationCancelRequest(?cid) : true <- acre.confirmCancel(?cid);
+conversationCancelRequest(?cid) : true <- acre.denyCancel(?cid);
Depending on whether the cancellation is agreed to or not, the agent that initiated the cancellation will have either a conversationCancelConfirmed
or conversationCancelFailed
event, so that it can take appropriate action in either case.
Debugging
ACRE makes it far easier for developers to observe the communication their agents are involved in. A Conversations tab is loaded into the Agent Factory debugger any time the ACRE Module is deployed in an agent. This allows you to filter ongoing conversations by the participating agent or by the underlying protocol.
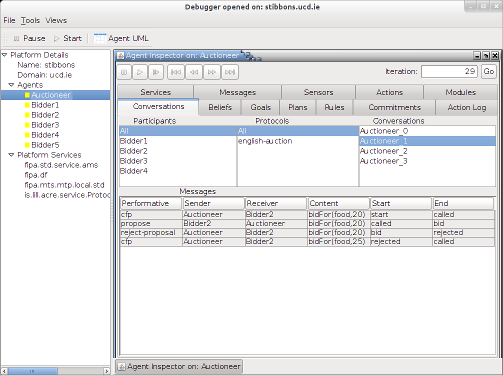